Remote devices (such as an Android device, an iPhone or even a simulator) will not have access to your local WCF service. You will need to know your Windows 10 workstation IP address on the local network. For the purpose of this example, assume that your workstation has the IP address 192.168.1.143. To get started with WCF, it might be easiest to just use the default SOAP format and HTTP POST (rather than GET) for the web-service bindings. The easiest HTTP binding to get working is 'basicHttpBinding'. Here is an example of what the ServiceContract/OperationContract might look like for your login service. The process of consumption of a WCF service hosted in IIS 5/6 is discussed below in detail. In addition, the discussion includes how to create proxy and console applications. Step 1 − Once a service is hosted in IIS, we have to consume it in client applications. Before creating the client application, we need to create a proxy for the service. Android did not provide any tool to help consuming SOAP web service. But as Android bundled with org.apache.http and org.json packages, it is relative simple to consume RESTful WCF services. The following sections describe the steps to create RESTfule WCF services and the Android client to consume the services.
- Download Wcf Client
- Wcf Service Android Client Manager
- Wcf Service Android Client Login
- Wcf Service Android Client Portal
This topic demonstrates how to enable a Windows Communication Foundation (WCF) service to authenticate a client with a Windows domain username and password. It assumes you have a working, self-hosted WCF service. For an example of creating a basic self-hosted WCF service see, Getting Started Tutorial. This topic assumes the service is configured in code. If you would like to see an example of configuring a similar service using a configuration file, see Message Security User Name.
To configure a service to authenticate its clients using Windows Domain username and passwords use the WSHttpBinding and set its Security.Mode
property to Message
. In addition you must specify an X509 certificate that will be used to encrypt the username and password as they are sent from the client to the service.
On the client, you must prompt the user for the username and password and specify the user’s credentials on the WCF client proxy.
To configure a WCF service to authenticate using Windows domain username and password
Create an instance of the WSHttpBinding, set the security mode of the binding to WSHttpSecurity.Message, set the
ClientCredentialType
of the binding to MessageCredentialType.UserName, and add a service endpoint using the configured binding to the service host as shown in the following code:Specify the server certificate used to encrypt the username and password information sent over the wire. This code should immediately follow the code above. The following example uses the certificate that is created by the setup.bat file from the Message Security User Name sample:
You can use your own certificate, just modify the code to refer to your certificate. For more information about creating and using certificates see Working with Certificates. Make sure the certificate is in the Trusted People certificate store for the Local Machine. You can do this by running mmc.exe and selecting the File, Add/Remove Snap-in... menu item. In the Add or Remove Snap-ins dialog, select the Certificates snap-in and click Add. In the Certificates Snap-in dialog select Computer account. By default the certificate generated from the Message Security User name sample will be located in the Personal/Certificates folder. It will be listed as 'localhost' under the Issued to column in the MMC window. Drag and drop the certificate into the Trusted People folder. This will allow WCF to treat the certificate as a trusted certificate when performing authentication.
To call the service passing username and password

Download Wcf Client
The client application must prompt the user for their username and password. The following code asks the user for username and password:
Warning
This code should not be used in production as the password is displayed while being entered.
Create an instance of the client proxy specifying the client's credentials as shown in the following code:
See also
Baudouin Giard
SoftFluent
September 2011
Summary: This article demonstrates how to consume mobile-enabled WCF services that were generated by CodeFluent Entities in an Android application.
Contents
I. Introduction
II. General Overview
III. The Mobile Application
This technical article illustrates how to consume, in an Android application, mobile-enabled WCF services as generated in the Generating Mobile-Enabled WCF Services Using CodeFluent Entities technical article.
This article will start by doing a brief overview of common mobile application design patterns, before an example implementation.
Please note that a complete working solution is provided with this example, so feel free to download and tour the code.
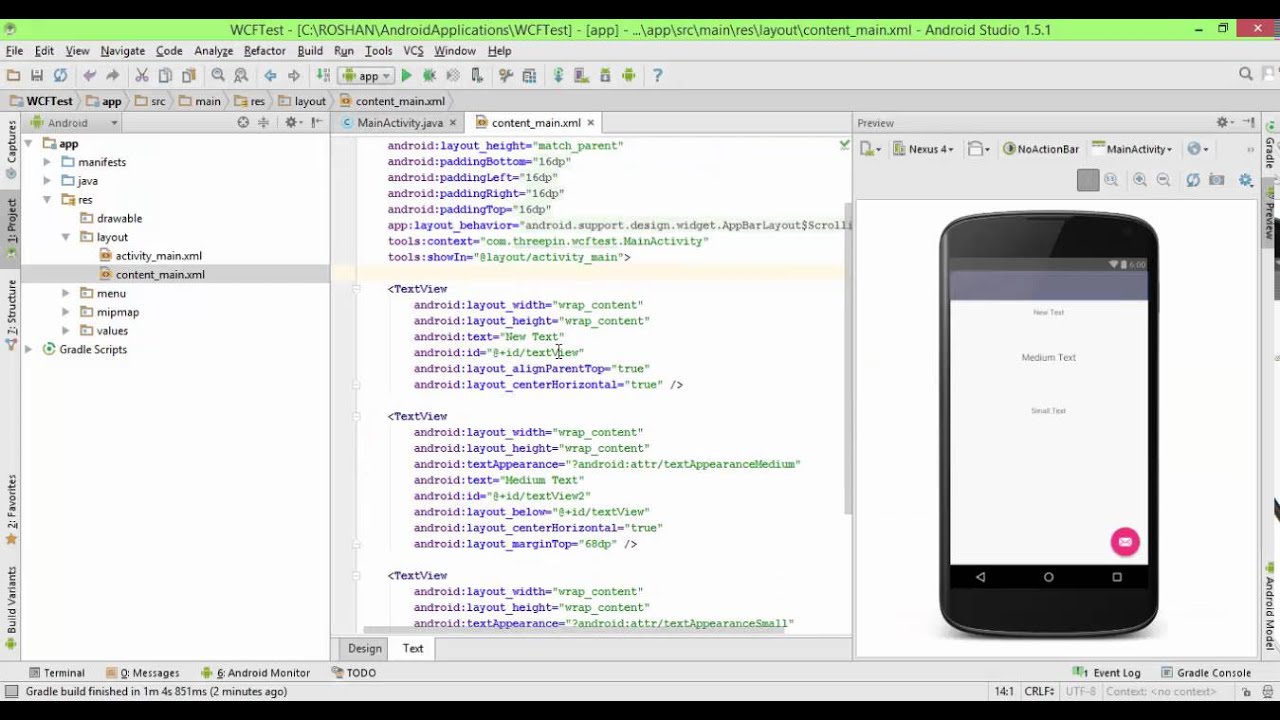
RESTful applications are amongst the most common application types in mobile environments; consequently it is easy to find documentation regarding this type of development on the internet.
The goal of our mobile applications is to show how to retrieve and how to create information on the server. Basically, in each application, we will:
- Connect to the Employee web service
- Display a list of all the employees of the company
- Allow the end-user to fill in a form matching the HolidayRequest entity
- Send this holiday request by connecting to the HolidayRequest web service.
A. Common mobile design patterns
Wcf Service Android Client Manager
If this is not your first mobile development you are probably already familiar with the typical design patterns for mobile platforms. Here are a few characteristics of a mobile app that differ from a desktop app and that a mobile developer must always keep in mind all along his work:
- the network might become unavailable at any time and queries might not come to an end,
- the application could be unexpectedly paused (e.g. by an incoming phone call, or by pressing the home button),
- a mobile CPU is slow and consumes a lot of battery life, so it must be solicited as less as possible,
- battery life is strategic and must be saved,
- data transfers must be avoided as much as possible, for battery savings as much as for billing savings,
- the application must scale on the largest panel of devices possible, so it must save memory to run on old phones.
In this article, I won’t necessarily respect all those patterns, since I’m not a confirmed mobile developer, and also in order to keep my code as easy to understand as possible. I will mainly focus on the communication part of the RESTful application, rather than mixing up things with advanced programming unrelated to the subject. You can see those developments as proof-of-concepts; it is up to you to implement all the right design patterns to make your enterprise-class business applications.
B. Generic RESTful design pattern
Here is what could be, in my opinion, a rather good design pattern for a RESTful application. I inspired from Virgil Dobjanschi’s Google I/O lecture. Dobjanschi explains that the intuitive way to implement a REST communication - create a worker thread within the UI thread - is a bad idea, since the UI thread might be interrupted anytime. He also gives some good ways to implement properly the REST architecture on a mobile device.
Here is the pattern I used:
1. Presentation layer
The presentation will be in charge of displaying our list of employees, let the user fill in a form corresponding to a holiday request, and trigger the upload of this request to our service.
The best way to do this is to implement a data binding between an in-memory model and the user interface.
2. Model layer
The model layer is in charge of keeping the application consistent and the code we develop as structured as possible. It allows manipulating the same structure of data on our mobile platform that the one we designed in CodeFluent Entities.
3. Persistence layer
The persistence layer is optional. Most of the time, we do not implement this layer on desktop smart-client applications because we assume that the network connection is permanent. In mobile phone development, even if a lot of applications are working this way, we should not go with this hypothesis for the many reasons that I exposed in the Common mobile design patterns section.
This layer is in charge on providing the application with intermediary persistence storage between the UI and the Data Access layer. When the application is killed, the data stays on the phone and can be instantly displayed at a next launch. This is the little “plus” that make some mobile applications better than others.
In our example, it would be especially good because our application downloads a list of the company’s employees, a data that is unlikely to change a lot: this means it doesn’t need to be downloaded each time the application is launched.
4. Data access layer
The Data Access layer is the part that interests us. This layer is in charge of getting the information remotely and converting it to model objects. It is also in charge of sending back to the service newly created objects. The Data Access layer may be itself divided in two sub-layers: a web client and a serialization processor.
The client will do the networking part and the processor will be in charge of serializing and deserializing the parameters and the results of the network transactions.
Wcf Service Android Client Login
If you want to have a better understanding on how to develop RESTful applications on mobile devices, I invite you to watch the video of the Google I/O lecture linked above (~60’) or have a look to the slides of this presentation.
I tried to stick the most I could to the first design pattern Virgil Dobjanschi presents in its Google I/O lecture:
The only difference is that in my case the Service is connected to the Content Provider instead of the Processor.
A. Presentation
In Android development, a presentation view is called an Activity. In my case, I configured the Android Virtual Device as a tablet, which comes with a 10” screen. It allows me to have only one Activity in my application.
Designed in the file res > layout > main.xml, the view matches our HolidayRequest entity definition:
From top to bottom in the UI we have:
- A refresh button
- A spinner containing the list of our employees
- A textbox to indicate the number of days of the requested vacation
- Two date pickers for the begin date and the end date
- A spinner to choose the type of vacation
- A textbox to write a comment
- A save button
The controller of this view is the MainActivity class of the com.softfluent.mobileholidays package. It just contains event handlers for the buttons “Refresh List” and “Send my query”. Those handlers trigger the web service and receive the results. It also contains a SimpleCursorAdapter ensuring the data binding between the employee content provider and the employee spinner list.
Wcf Service Android Client Portal
B. Model
I had to create Java classes for each of my entities. I put them in the package com.softfluent.mobileholidays.model. They are really simple classes matching the model we designed in CodeFluent Entities, except for the relations (don’t forget what I mentioned above about lazy-loading).

Tip: You can use your SoftFluent.MobileHolidays.ConsoleApp project to see what a serialized entity instance looks like. |
However, we have to add Android-specific code to this base model.

According to the diagram above we will create a HolidayRequest from the Activity and pass it down to the Service, in Android we need it to implement the Parcelable interface which is a form of binary serialization.
In order to respect the chosen pattern, we will implement the optional persistence layer. We will use an Android ContentProvider to store our Employee objects. We must add a nested Employees class to our Employee class, implementing the BaseColumns interface:
Employee.java | Copy Code |
---|
See the Content Providers section of Android developer guide if you are not familiar with data storage on Android.
C. Persistence
Like I said, we will implement a persistence layer in our Android application. Android provides the developer with a generic concept of content providers that give a standard way to retrieve, store and manipulate data from applications.
I chose to implement a SQLLite Database based Content Provider to store the Employee list because this is the most common way to do it. The “employees” spinner in the Activity is connected to this Content Provider by a SimpleCursorAdapter which provides a binding between the view and the data.
It represents a lot of code, but pretty much everything is generic and easily reusable from one model to another just by changing the name of the fields. I invite you to consult the code of the file: EmployeeContentProvider.java. This a basic implementation of a Content Provider, such as those you can easily find on the web.
D. Data Access
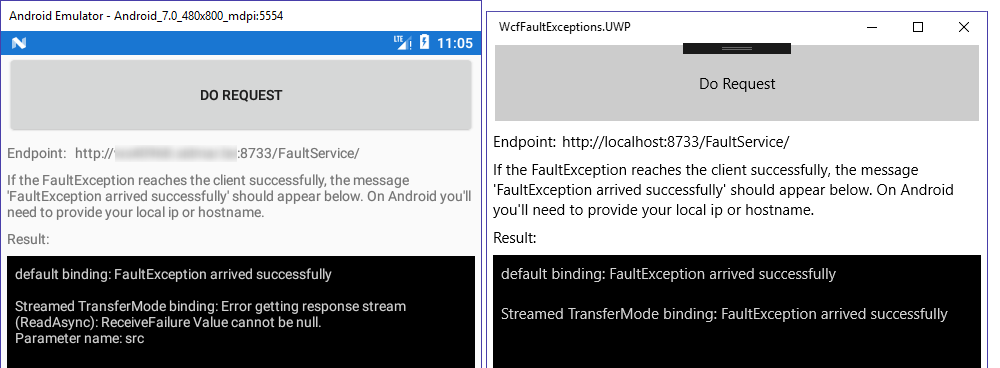
Here is the part in which we will consume our services.
The Service Helper is just a class implementing a few static methods to create an Intent object to start the Service. A Service is, on Android, a long-running background operation.
Our Service inherits the IntentService class, which is a base class designed for services like ours.
WebService.java | Copy Code |
---|
Our Service receives its parameters in its “extras” Bundle:
- A ResultReceiver to which it will send the result of the web service transaction,
- Depending on the 'action' specified in the Intent, a HolidayRequest object.
Note that our service is the part of our model dealing with the Employees Content Provider. On the diagram, it is specified that the Processor would do it. It just made more sense to me at the time I coded it not to delegate the storage operations to the processor: it makes the processor less complicated, and easier to replace if we need someday to change the serialization format.
The Processor class is just a set of static methods, each corresponding to a service operation. The Processor delegates the network interaction to the RestClient class, and just serializes and deserializes the objects. The JSON serialization is handled by the Google Gson library.
Note that we created a custom JSONSerializer for the dates, because the default serialization of dates by Gson did not match the one used by the services in the first place (remember that a date is not a standard type in Json):
JSONSerializer<Date> | Copy Code |
---|
Last, the most important: the Rest client. I used the Apache Http Client, as recommended in the Google I/O lecture. Note this is standard Android development: the org.apache.http package is embedded in Android SDK.
This is a very simple implementation. A better way to do it would be to create a RestClient[EntityName] class for each entity, implementing methods corresponding to each method exposed by the web services.
Yet, this illustrates how to consume WCF services generated by CodeFluent Entities in an Android application.
Consuming Generated WCF Services in Windows Phone
Consuming Generated WCF Services in iOS
